LEARNING OBJECTIVES
Understand and use suitable loops including count and condition controlled loops
SUCCESS CRITERIA
Success Criteria:
-
Use a condition-controlled loop (while) to repeat instructions based on a certain condition.
-
Use a count-controlled loop (for) to repeat instructions a fixed number of times.
3.1 Programming Techniques
Learners should have studied the following:
-
3.1.1 how to identify and use variables, operators, inputs, outputs and assignments
-
3.1.2 how to understand and use the three basic programming constructs used to control the flow of a program: Sequence; Selection; Iteration
-
3.1.3 how to understand and use suitable loops including count and condition controlled loops
-
3.1.4 how to use different types of data, including Boolean, string, integer and real, appropriately in solutions to problems
-
3.1.5 how to understand and use basic string manipulation
-
3.1.6 how to understand and use basic file handling operations:
-
open
-
read
-
write
-
close
-
-
3.1.7 how to define and use arrays (or equivalent) as appropriate when solving problems
-
3.1.8 how to understand and use functions/sub programs to create structured code.
CODING TIP
Common Errors:
Off-by-one error occurs when we have "<=" instead of "<" when we are checking the expression in the loop.
The example below will illustrate the problem.
for count in range(0, 5):
print( "I am in loop number: " + str (count))
The output will be:
I am in loop number: 0
I am in loop number: 1
I am in loop number: 2
I am in loop number: 3
I am in loop number: 4
You csn see that the numbers output range from 0-4 and not 0-5.
Challenge:
How could you edit the code to fix this?
Write a program that outputs the word computing 15 times.
Use the variable i inside the loop.
Example:
1 Computing
2 Computing
3 Computing...
Count Controlled
TASK 1
Create a program which will count down from 10 to 0, indicating how long there is to go before time runs outs. When time runs out it should display a suitable message.
Example:
"You have ..... seconds remaining"
Count Controlled
TASK 2
Task 1 & 2 too easy??
Write a program that will ask the user for a message and the number of times they want that message displayed. Then output the message that number of times.
Example:
User inputs message....Mrs N is the best!
User inputs no. of times....14
Output:
1 Mrs N is the best!
2 Mrs N is the best!
3 Mrs N is the best!
4 Mrs N is the best!
5 Mrs N is the best!
...
Count Controlled
STRETCH & CHALLENGE
CODING TIP
Common Errors:
Forgetting to advance your control variable
Make sure that the variable involved in the test changes in the body of the loop.
Example:
mycount = 1
while mycount <= 100:
print mycount
Challenge:
Predict what might happen if this program were to run.
How could you edit the code to fix this?
Wrong incrementing
If you want to decrement, make sure that you do not automatically increment
Example:
mycount = 100
while mycount > 0:
print mycount
mycount += 1
Challenge:
Predict what might happen if this program were to run.
How could you edit the code to fix this?
Write a program that asks for a password and keeps asking until the correct password,
apple is entered and then says Accepted.
Condition Controlled
TASK 1
Create a program which iterates the message "Please enter your name: "
until they enter the word name.
You must use the while function to control this.
Condition Controlled
TASK 2
Task 1 & 2 too easy??
Write a program to take in values from the user until user enters 'done', then output the highest and the lowest values.
Example:
User enters...21
User enters...100
User enters...12
User enters...57
User enters...3
User enters...done
Highest...100
Lowest...3
Condition Controlled
STRETCH & CHALLENGE
3.1.3 count and condition controlled loops
STARTER
Let’s remind ourselves of the different types of iteration that can occur in a program. Iteration is a much more effective way of repeating the same code over and over again. Complete the tasks below independently, then peer assess.
Stretch & Challenge:
When should a programmer use
a for loop instead of a while
loop?
-
to understand and use suitable loops including count and condition controlled loops
Iteration is the process of repeating sections of a program to achieve a particular target or goal.
Computer programs can use different types of loops:
-
infinite loops - repeat forever
-
count-controlled loops - repeat a set amount of times
-
condition-controlled loops - repeat until something happens
COUNT-CONTROLLED LOOPS
Count-controlled loops are used to make a computer do the same thing a specific number of times.
The count-controlled loop can be described as a FOR loop. The program repeats the action FOR a number of times.
for i in range (x,y):
do something
Count-controlled repetition requires:
-
control variable (or loop counter) i
-
starting value of the control variable x
-
increment (or decrement) by which the control variable is modified each iteration through the loop y
A count-controlled repetition will exit after running a certain number of times. The count is kept in a variable called an index or counter. When the index reaches a certain value (the loop bound) the loop will end.
In the loop for i in range(x,y) x is the starting value of i and y is the one above
what it will get to. So above it runs with i equal to 0,1,2,3 and 4. Traditionally loops tend to use the variables i,j and k and you may see lots of code that conforms to this convention. There is, however, no reason why you can’t use any other variable name especially if it makes your code more readable.
Here is an example using pseudocode:
INITIALIZE count to 0
FOR as long as “count” is in the range of 0 to 5
OUTPUT “I am in loop number: ” and the value contained in “count”
INCREMENT “count” by 1
Here is an example using flowcharts:

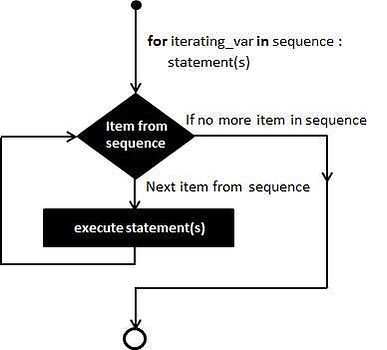
FOR LOOPS in python...

WE ARE LEARNING..
CONDITION-CONTROLLED LOOPS
A program could be made more intelligent by programming it to avoid hazards. For example, if the robot vehicle is 3 cm from the edge of the table and you tell it to move forwards 5 cm, it will drive off the edge of the table. To stop this from happening, you might write a condition-controlled loop like this:
move forward
repeat until (touching table edge)
Condition-controlled loops can be used to add a high degree of intelligence to a computer system.
The condition-controlled loop can be described as a WHILE loop. The program repeats the action WHILE a condition is true (or false as the case may be).
Whether the condition is met or not is checked at the beginning of the loop. If the condition is 'true' it repeats, if not then the code is not executed.
while (condition==TRUE):
do something
Condition-controlled loops appear in many ways, depending on the task to be completed.
Here is an example using flowcharts:
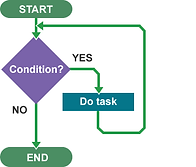
1.
WHILE LOOP
Example:
words =input("Say Something: ”)
while words != “bye”:
print (words)
words = input("Say Something: ”)
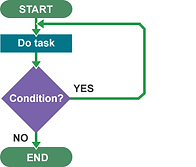
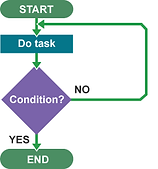
2.
DO WHILE LOOP
Example:
count = 1
do
print(count) count+=1
while count <4
3.
REPEAT UNTIL LOOP
Example:
count = 1
repeat
print(count) count+=1
until count >3
WHILE LOOPS in python...
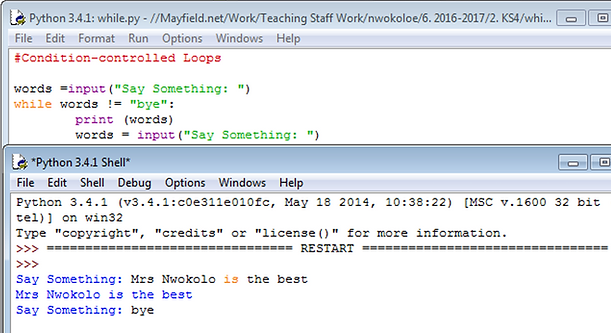