LEARNING OBJECTIVES
Define and use arrays (or equivalent) as appropriate when solving problems
SUCCESS CRITERIA
Success Criteria:
-
Choose a colour scheme
-
Create suitable text boxes
-
Decide on content layout
3.1 Programming Techniques
Learners should have studied the following:
-
3.1.1 how to identify and use variables, operators, inputs, outputs and assignments
-
3.1.2 how to understand and use the three basic programming constructs used to control the flow of a program: Sequence; Selection; Iteration
-
3.1.3 how to understand and use suitable loops including count and condition controlled loops
-
3.1.4 how to use different types of data, including Boolean, string, integer and real, appropriately in solutions to problems
-
3.1.5 how to understand and use basic string manipulation
-
3.1.6 how to understand and use basic file handling operations:
-
open
-
read
-
write
-
close
-
-
3.1.7 how to define and use arrays (or equivalent) as appropriate when solving problems
-
3.1.8 how to understand and use functions/sub programs to create structured code.
VIDEO
CODING TIP
REMEMBER:
Lists can only store one data type.
Normally, the maximum size of the array is declared.
e.g. Names(9)
CODING TIP
REMEMBER:
Computers count from zero, so the first number in the array is always counted as 0, the 15th number will be counted as 14. The last element in an array with n elements will have the index n-1.
Write a program that keeps asking for names until the word END is entered at which point it prints out the list of names.
LISTS
TASK 1
Create a program which takes in a shopping list of 10 items and sorts them alphabetically.
You will need to use the .sort() function.
Research this, if you havent come across it before.
LISTS
TASK 2
Task 1 & 2 too easy??
Write a program that prints out all the prime numbers below 10,000.
Hint: you will need to use % which gives the remainder of a division operation.
So x=11%5, would give 1 as 11/5=2 remainder 1.
Go to Page 3.1.1 [Operators] for help on this.
Condition Controlled
STRETCH & CHALLENGE
3.1.7 definING and usING arrays
STARTER
Design a wix page template for Computer Science.
-
how to define and use arrays (or equivalent) as appropriate when solving problems
ARRAYS
The "Letterbox" Analogy
How are they used?
Imagine you were making a game and you wanted to store player names and their scores.
You would need to write some code to tell the program to store this information.
When arrays are created, the program needs to know their size. It needs to know how many player names and scores you want to collect. When an array or any variable is created in a program this is called declaring. For example, if you created two arrays which could store five pieces of data each for player scores and player names, they would be declared with the following:
-
gameScores[5]
-
playerNames[5]


Two-dimensional arrays
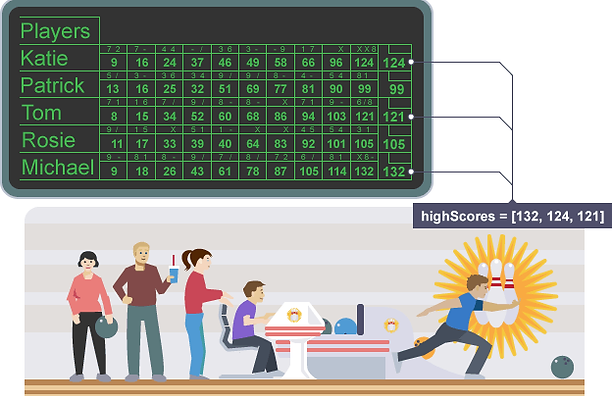
A one-dimensional array can be seen as data elements organised in a row. A two-dimensional array is similar to a one-dimensional array, but it can be visualised as a grid (or table) with rows and columns. << Like in the Bowling example above.
For example, a nine-by-nine grid could be referenced with numbers for each row and letters for each column. A nine-by-nine, two-dimensional array could be declared with a statement such as:
game [9][9]
Many games use two dimensional arrays to plot the visual environment of a game. Positions in a two dimensional array are referenced like a map using horizontal and vertical reference numbers. They are sometimes called matrices.
We are not limited to making one and two-dimensional arrays. We can also make three-dimensional arrays as well. Many games (such as Minecraft) use three-dimensional arrays to model an environment.
INDEXES
An index is used to point at a data element within an array .
For the gameScores array mentioned on page 2 there are five data elements:
gameScore = (124, 99, 121, 105, 132)
Each can be referenced according to their position in the array: 0, 1, 2, 3, 4.
In arrays, the first data element is identified by 0, the second is 1, the third is 2, etc.
To refer to the first element in the gameScores array, we would need to ask for ‘Index zero’ of gameScores. The following statement would be written:
gameScores[0]
This would return the value ‘940’.
LISTS
A Python array is a little bit different to arrays in other programming languages in that it uses something called 'list' instead of array. Python 'lists' offer more flexibility than arrays as they can contain different types of data and their length can be varied.
There are advantages and disadvantages of using both types.
Advantages of Lists!
It is often convenient to use a list where you do not need to know the data type or length.
Advantages of Arrays!
However, arrays are a more direct way of accessing data in memory so they are much faster and more efficient.
----- As we are programming in Python here at Mayfield,
we will refer to Lists -----
LISTS in python...
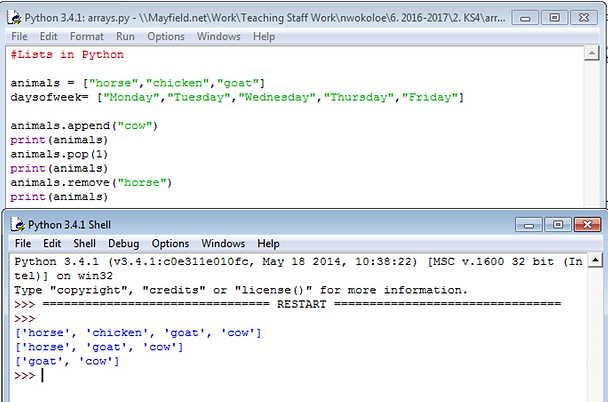
To add entries to the list we then call the append function.
Example:
animals.append("cow")
print(animals)
Output:
['horse', 'chicken', 'goat', 'cow']
To remove an item from the list we use the pop method if we want to remove by index.
Example:
animals.pop(1)
Output:
['horse', 'goat', 'cow']
If we know the item we want to remove we can use the remove function which deletes the
first instance of it.
Example:
animals.remove("horse")
Output:
['goat', 'cow']
Learn more list functions here: http://www.thegeekstuff.com/2013/08/python-array/